As I have mentioned on the main blog, this is under a Creative Commons Share-Alike license. You may use, alter, and distribute the contents of this e-book, but you need to give credit and distribute your code under the same or similar license.
Programming can be challenging from time to time, but it is pretty easy if you break it down. Sketches (what Arduino programs are called) are usually short and to the point which makes them even easier to program.
IMPORTANT: Spelling and capitalization matters! Incorrectly capitalize or spell a variable, function, library, ect, and your code won't work.
Basics of Programming the Arduino
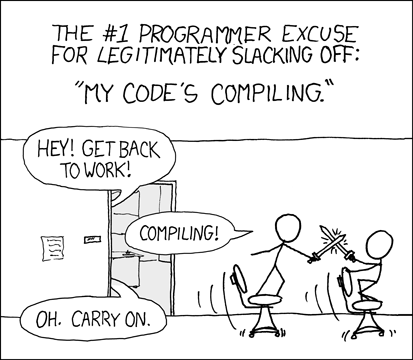
Here is a simple sketch that turns the onboard LED on and off every second.
#define LED 13 //every time the program sees "LED"
//it will replace it with "13"
//Pin 13 is the onboard LED
int wait = 1000;
void setup() {
// Sets pin 13 to OUTPUT
pinMode(LED, OUTPUT);
}
void loop() {
digitalWrite(LED, HIGH); // set the LED on
delay(wait); // wait for a second
digitalWrite(LED, LOW); // set the LED off
delay(wait); // wait for a second
}
You might notice a couple of "odd" things here:
pinMode() is used to choose whether a pin is INPUT or OUTPUT. As we only look to set it one time, it was placed in the setup() function
digitalWrite() is used to apply 0V to a pin (LOW) or 5V to a pin (HIGH).
delay() will wait the number of milliseconds you put inside.
int is short for "integer" and is use to define a variable. There are many different types of variables, all of which have pros and cons. See here for more info on all of the data types.
As we go over more material in the Workshops, this section will grow to cover it.